ListView¶
Screenshot¶
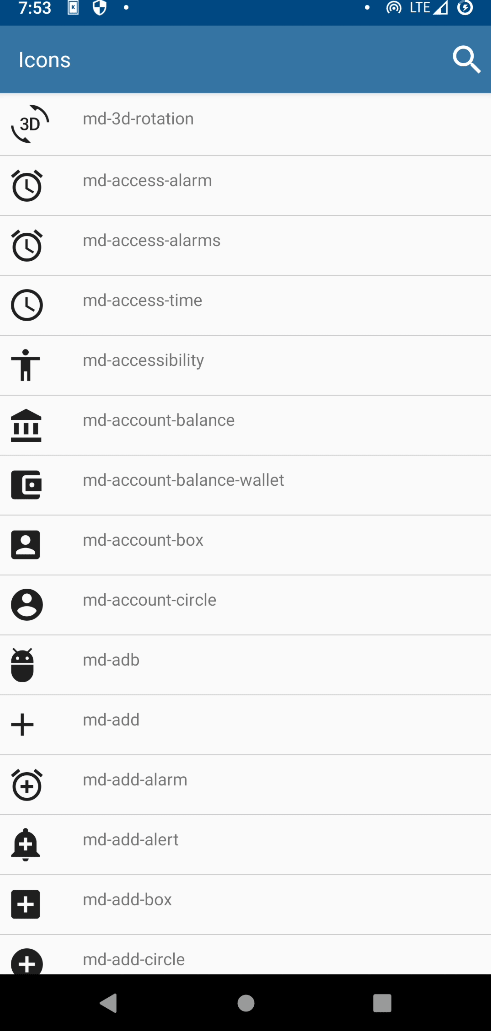
Example¶
"""
Demonstrates using a ListView
for this to work you must first install enaml-native-icons with
conda config --add channels codel
enaml-native install enaml-native-icons
# Then rebuild
enaml-native run-android
"""
from atom.api import Atom, Str, List, Bool
from enamlnative.core.api import *
from enamlnative.widgets.api import *
import iconify
from iconify import material
iconify.install()
class AppState(Atom):
_instance = None
search = Str()
data = List()
show_search = Bool()
def _default_data(self):
return list(material.ICONS.keys())
@classmethod
def instance(cls):
""" Use this to access the state """
if cls._instance is None:
cls._instance = cls()
return cls._instance
def __init__(self):
""" Make it a singleton """
if AppState._instance is not None:
raise RuntimeError("Only one AppState should exist!")
super(AppState, self).__init__()
enamldef ContentView(LinearLayout): drawer:
attr state = AppState.instance()
orientation = 'vertical'
AppBarLayout:
height = "wrap_content"
width = 'match_parent'
Toolbar:
Flexbox:
justify_content = "space_between"
align_items = "center"
TextView:
text = "Icons"
visible << not state.show_search
text_color = "#fff"
text_size = 18
clickable = True
EditText:
width = 'match_parent'
visible << state.show_search
placeholder = "Filter"
text_color = "#fff"
editor_actions = True
text := state.search
editor_action :: state.show_search = False
Icon:
text = "{md-search}"
visible << not state.show_search
padding = (10, 10, 10, 10)
text_size = 32
text_color = "#fff"
clickable = True
clicked :: state.show_search = True
ListView: list_view:
items << [s for s in state.data
if not state.search or state.search in s]
Looper:
iterable = range(30)
ListItem:
attr icon << item or ''
LinearLayout:
orientation = 'vertical'
Flexbox:
align_items = 'center'
padding = (10, 10, 10, 10)
Icon:
text << "{%s}"%icon
text_size = 32
width = 50
TextView:
text << "{}".format(icon)
padding = (10, 0, 10, 10)
View:
background_color = '#ccc'
height = 1
Declaration¶
- class enamlnative.widgets.list_view.ListView(parent=None, **kwargs)[source]¶
Bases:
enamlnative.widgets.view_group.ViewGroup
A widget for displaying a large scrollable list of items.
- items¶
List of items to display
- fixed_size¶
use this setting to improve performance if you know that changes in content do not change the layout size of the RecyclerView
- arrangement¶
Layout manager to use
- orientation¶
Orientation
- span_count¶
Span count (only for grid and staggered)
- proxy¶
A reference to the ProxyLabel object.
Android Implementation¶
- class enamlnative.android.android_list_view.AndroidListView[source]¶
Bases:
enamlnative.android.android_view_group.AndroidViewGroup
,enamlnative.widgets.list_view.ProxyListView
An Android implementation of an Enaml ProxyListView.
- widget¶
A reference to the widget created by the proxy.
- adapter¶
Reference to adapter
- layout_manager¶
Layout manager
- list_items¶
List items
- item_mapping¶
List mapping from index to view
No iOS implementation found.