Dialog¶
Screenshot¶
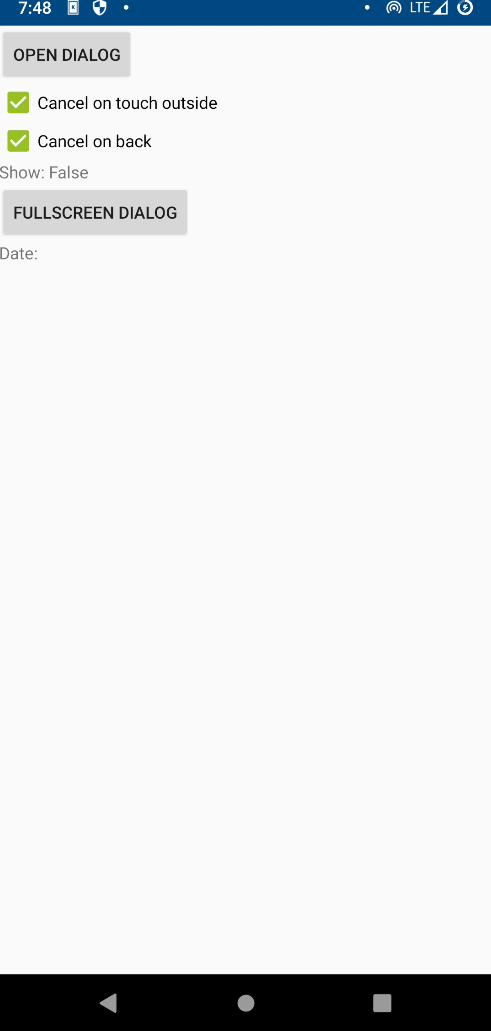
Example¶
from enamlnative.widgets.api import *
from enamlnative.android.app import AndroidApplication
enamldef ContentView(Flexbox):
flex_direction = "column"
Button:
text = "Open dialog"
clicked :: dialog.show = True
#: Prevent cancelling without pressing a button
CheckBox:
text = "Cancel on touch outside"
checked := dialog.cancel_on_touch_outside
CheckBox:
text = "Cancel on back"
checked := dialog.cancel_on_back
TextView:
text << "Show: {}".format(dialog.show)
Dialog: dialog:
Flexbox:
flex_direction = "column"
justify_content = "space_between"
padding = (20, 20, 20, 0)
TextView:
text = "Are you sure you want to delete?"
font_family = "sans-serif-medium"
TextView:
text = "This operation cannot be undone."
Flexbox:
justify_content = "space_between"
margin = (0, 20, 0, 10)
Button:
flat = True
text = "Ok"
clicked ::
print("ok!")
dialog.show = False
Button:
flat = True
text = "Cancel"
clicked :: dialog.show = False
Button:
text = "Fullscreen dialog"
clicked :: modal.show = True
TextView: date_text:
text = "Date:"
Dialog: modal:
#: Fullscreen style
style = "@style/Theme.DeviceDefault.Light.NoActionBar.Fullscreen"
#: Activity style (full screen but keep the action bar)
#style = "@style/ActivityDialog"
Flexbox:
flex_direction = "column"
CalendarView:
date ::
date_text.text = "Date: {}".format(change['value'])
modal.show = False
TextView:
text = "Pick a date or press back to exit"
Declaration¶
- class enamlnative.widgets.dialog.Dialog(parent=None, **kwargs)[source]¶
Bases:
enaml.widgets.toolkit_object.ToolkitObject
A popup dialog that may contain a view.
- cancel_on_back¶
Sets whether this dialog is cancelable with the BACK key.
- cancel_on_touch_outside¶
Sets whether this dialog is canceled when touched outside the window’s bounds.
- key_events¶
Listen for key events
- key_pressed¶
Key event
- title¶
Set the title text for this dialog’s window.
- show¶
Start the dialog and display it on screen (or hide if False)
- style¶
Dialog style using the @style format (ex. @style/Theme_Light_NoTitleBar_Fullscreen
- proxy¶
A reference to the proxy object.
- popup()[source]¶
Show the dialog from code. This will initialize and activate if needed.
Examples
>>> enamldef AlertDialog(Dialog): dialog: attr result: lambda text: None TextView: text = "Are you sure you want to delete?" Button: text = "Yes" clicked :: dialog.show = False dialog.result(self.text) Button: text = "No" clicked :: dialog.show = False dialog.result(self.text) def on_result(value): print("User clicked: {}".format(value)) AlertDialog(result=on_result).popup()
Notes
This does NOT block. Callbacks should be used to handle click events or the show state should be observed to know when it is closed.
Android Implementation¶
- class enamlnative.android.android_dialog.AndroidDialog[source]¶
Bases:
enamlnative.android.android_toolkit_object.AndroidToolkitObject
,enamlnative.widgets.dialog.ProxyDialog
An Android implementation of an Enaml ProxyDialog.
- dialog¶
A reference to the widget created by the proxy.
No iOS implementation found.